The Modern Web
Websites have advanced significantly since their inception a decade ago. We began with simple HTML and CSS-based webpages. Then came JavaScript, which completely changed the web’s functionality. Because of JavaScript frameworks, which made single-page applications (SPAs) a reality, web technologies have progressed significantly since then.
Today, we can create websites that only load the code files from the server once and never reload them again, even when the user navigates to different pages. What is the mechanism behind this?
If you’ve ever desired to work as a full-stack web developer, you’ve probably heard of the MERN Stack. But don’t worry if you haven’t.
What exactly is it?
What does MERN stand for?
How can you get started as a MERN stack developer?
I’m here to help you understand it and expose you to one of the most popular web development tech stacks, as well as provide you with a detailed roadmap and tools. Do you have any expectations? Let’s get started with the basics.
What is the MERN Stack
The MERN stack full form includes MongoDB, ExpressJS, ReactJS, and NodeJS, allows you to create feature-rich single-page web apps using only one programming language, JavaScript. One of the main reasons why the MERN stack is so popular, according to several developers, is because of this. You may handle both the front and back ends of your web application using a single language.
Let’s delve deeper into the MERN stack technologies that we have a fundamental knowledge of. Let’s start with MongoDB.
MongoDB
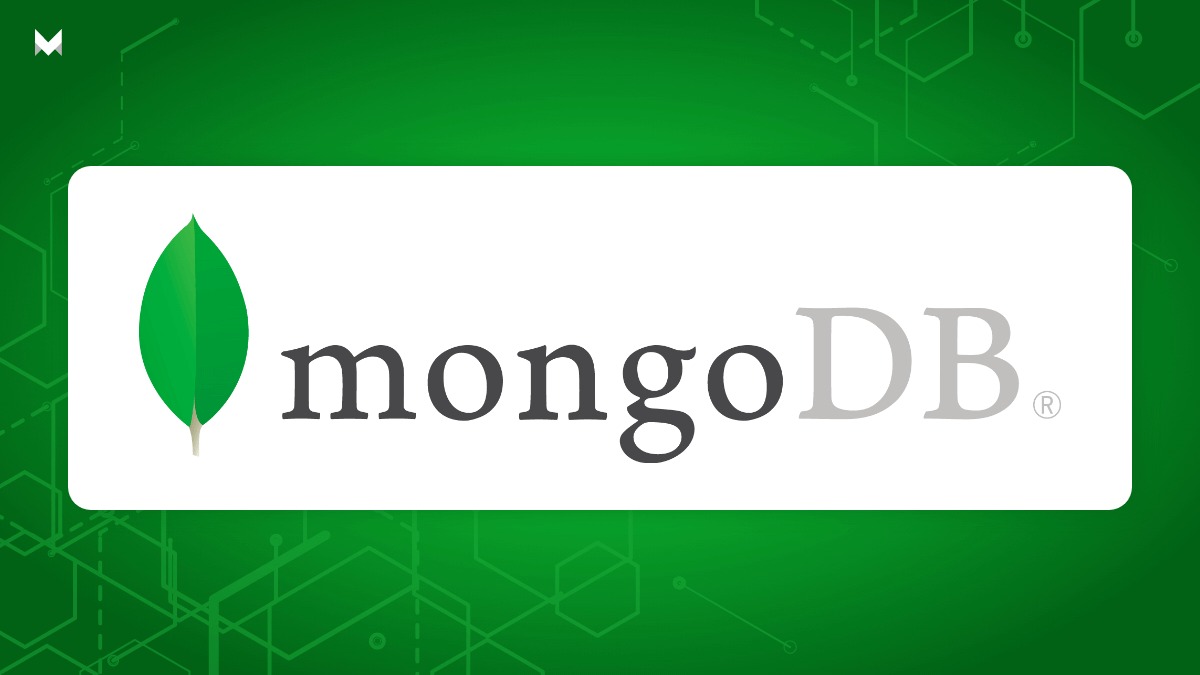
MongoDB is a NoSQL database that stores data as documents that are made up of key-value pairs, similar to JSON or JavaScript Object Notation. Too much jargon, wow, wow, Don’t be concerned! Let’s break down the above statement into smaller parts.
I’ll start with SQL vs. NoSQL. Tables are used to hold data in SQL databases. Tables are simply a tabular collection of data organised by rows and columns. It’s time to put your ideas on paper!
The following is an example of MongoDB data:
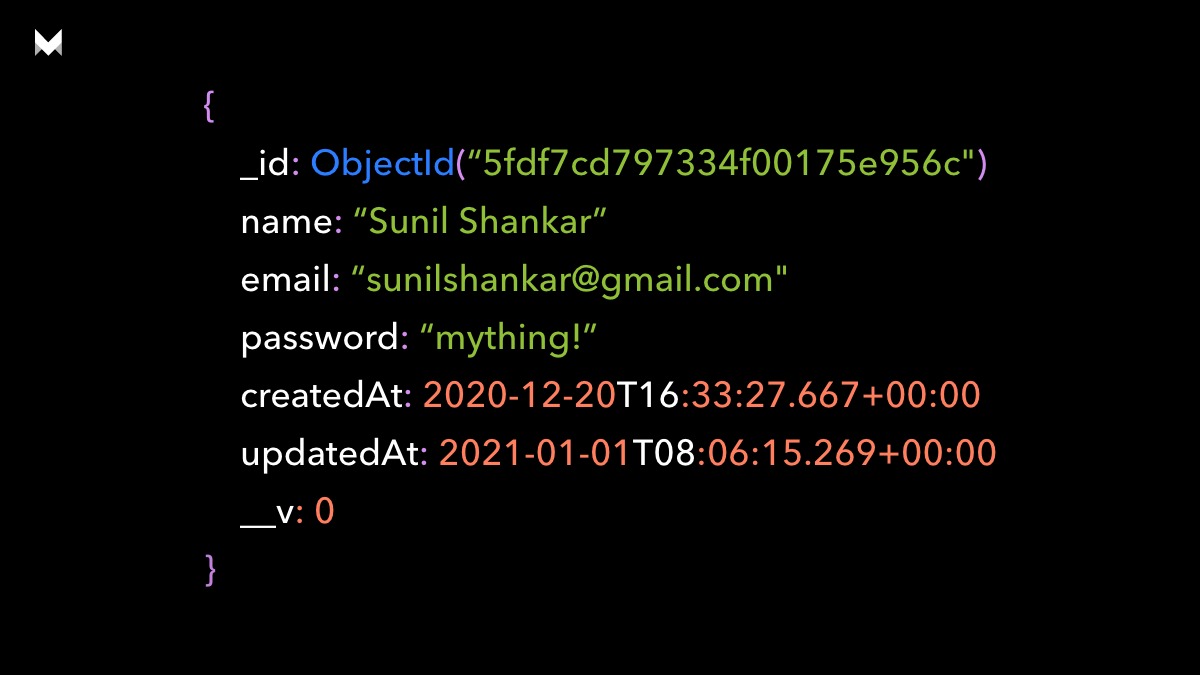
Here’s how data saved in MySQL, a SQL-based database, appears:
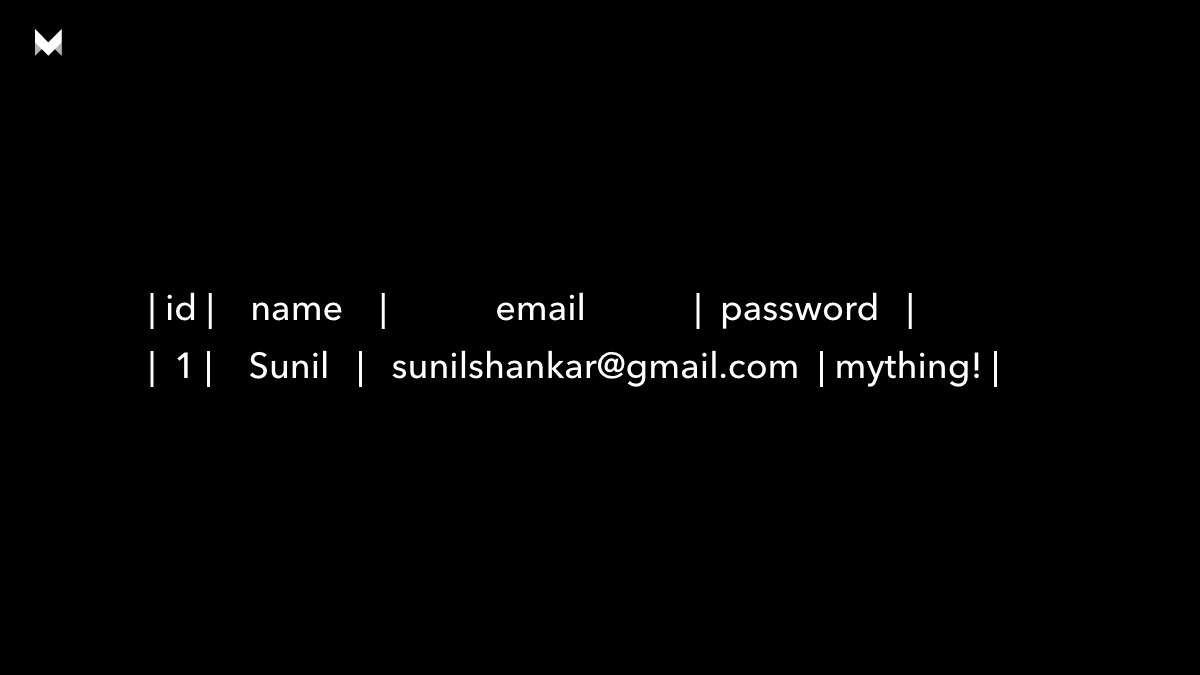
MongoDB excels in so many areas that it’s a fantastic pick for your forthcoming projects. Here are a few examples:
- Indexing provides high performance.
- Model schemas that are dynamic
- Data is distributed across several servers, resulting in high scalability.
- Ability to store geospatial data through GeoJSON
- Auto replication
And there’s a lot more!
Okay, but how will we save our data in a MERN stack development web application using MongoDB? While we may use the mongodb npm package, using an ODM or Object Data Modelling framework like mongoose is more convenient.
If I were to make a list of things you should understand about MongoDB for creating full stack web apps, it would include:
- Setting up a MongoDB Atlas database on your local machine or on the cloud
- Models and schemas are created.
- Use the database to perform CRUD (Create, Read, Update, and Delete) actions.
Bonus points are awarded for:
- Using references to connect two related models
- Understanding the pre- and post-hooks of mongoose
- Validation of Mongoose data
ExpressJS
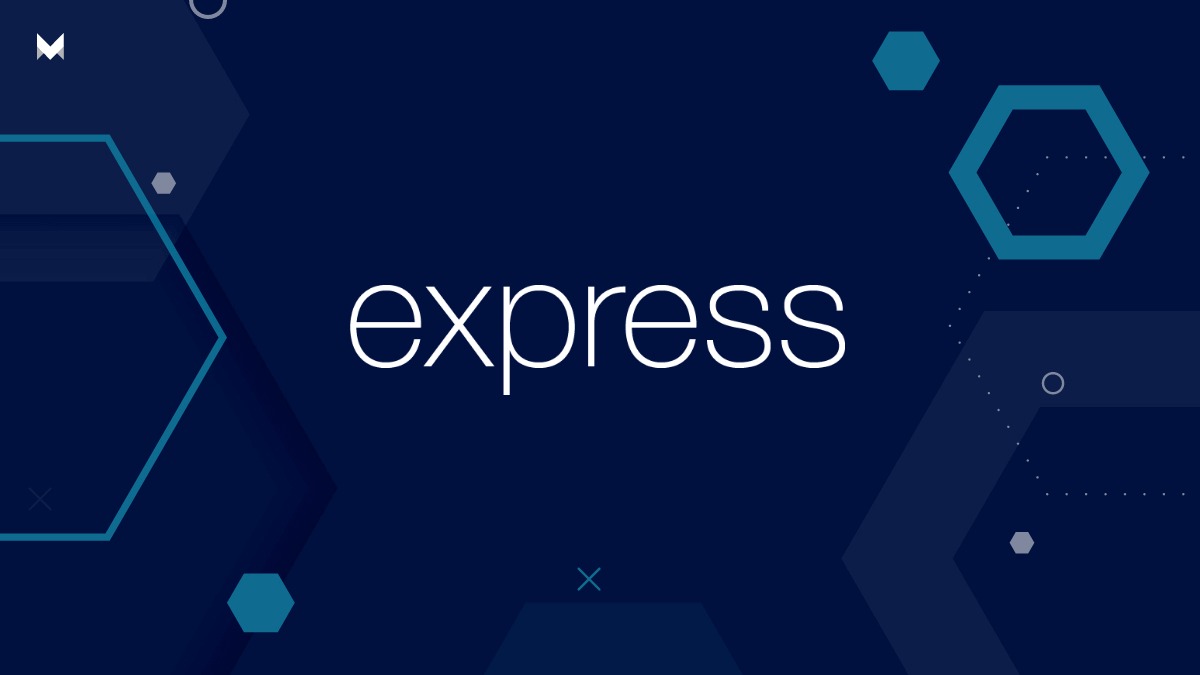
Before we move on to ExpressJS, let’s have a look at what it is, why we use it, and whether or not we need it (spoiler alert: we don’t!). Express is the most widely used NodeJS web application framework. Express’s purpose in MERN stack development apps is to handle our backend API server, which will be used to fetch data from a database via our React frontend.
In simplest terms, Express is used to listen to a certain port on our server for requests from the user, or frontend. For each endpoint that the user visits, we may construct various routes. Here’s an illustration to help you understand what I’m talking about:
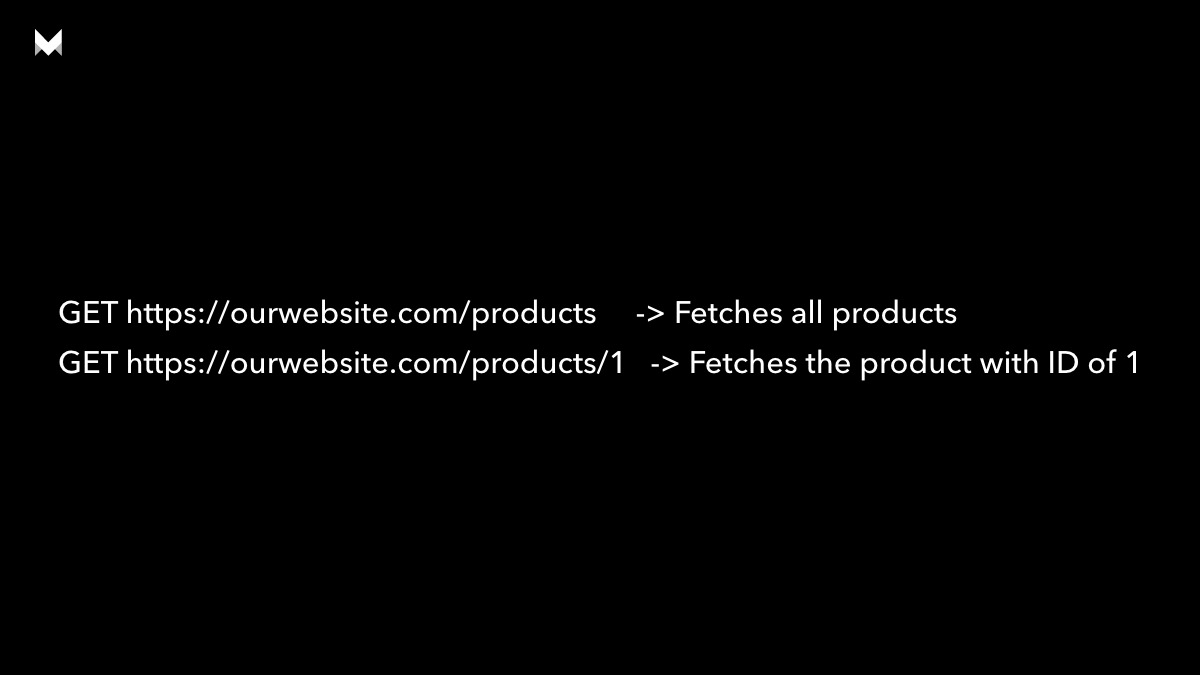
We create and implement the routes as programmers to receive the correct data from the correct endpoint. Express enables us to accomplish this rapidly. Remember how I claimed Express wasn’t strictly necessary? That’s because we can construct the routes I described before using the core http module that NodeJS offers. Why don’t we make advantage of that? Because Express improves the development experience in general.
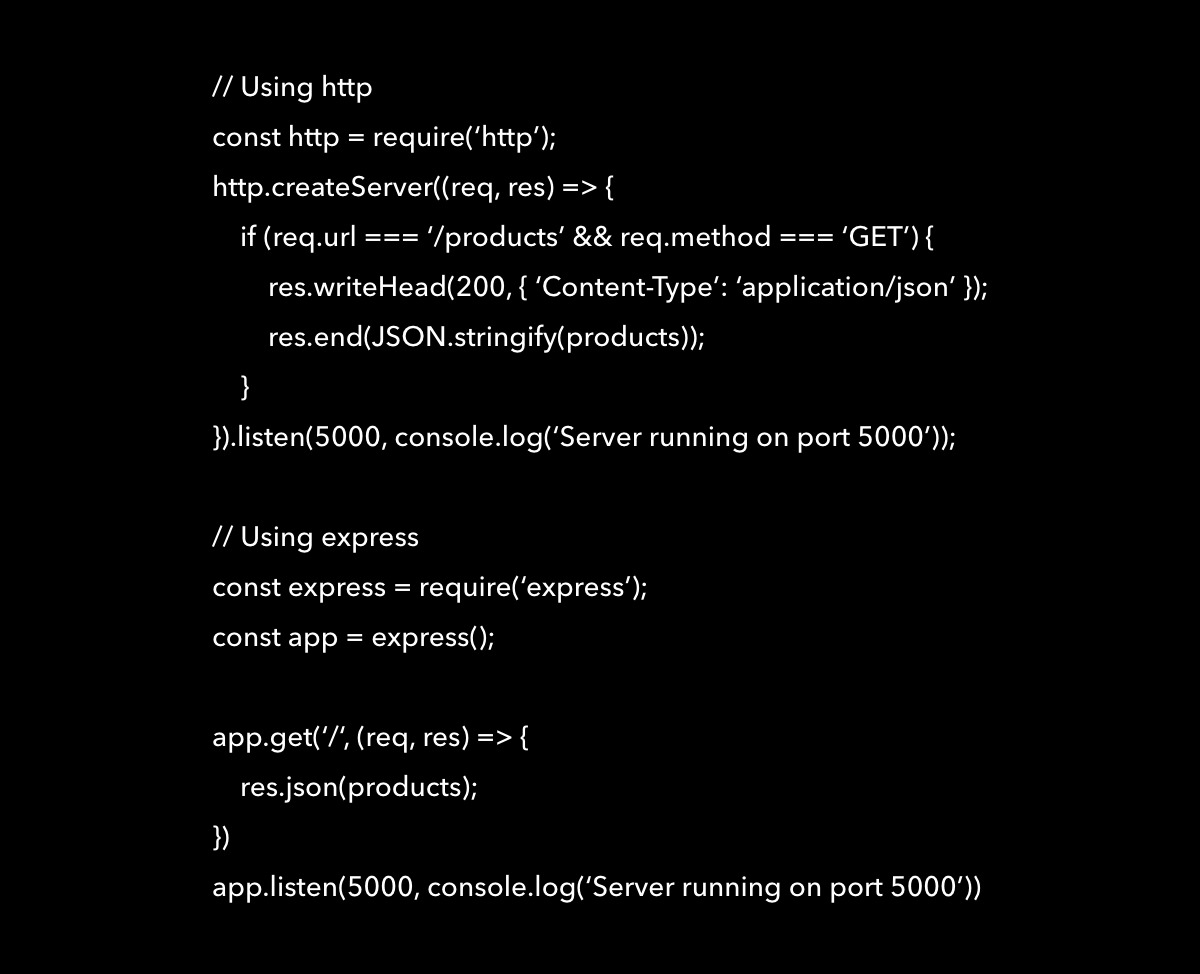
This was only an example, and it was a very simple one at that. Express code is considerably easier to understand and write than traditional code. Now, as a dedicated developer, here are some things you should know about Express:
Configuring an express server to listen on a certain port
Create GET, POST, PUT, and DELETE routes/endpoints for data CRUD operations.
Reading JSON form data supplied over express from the frontend.
json() middleware
Using Express to set up an ODM like Mongoose
Extra credit for:
Separating logic into separate files, such as controllers, routes, and models
Developing bespoke middlewares to deal with faults and other issues
ReactJS
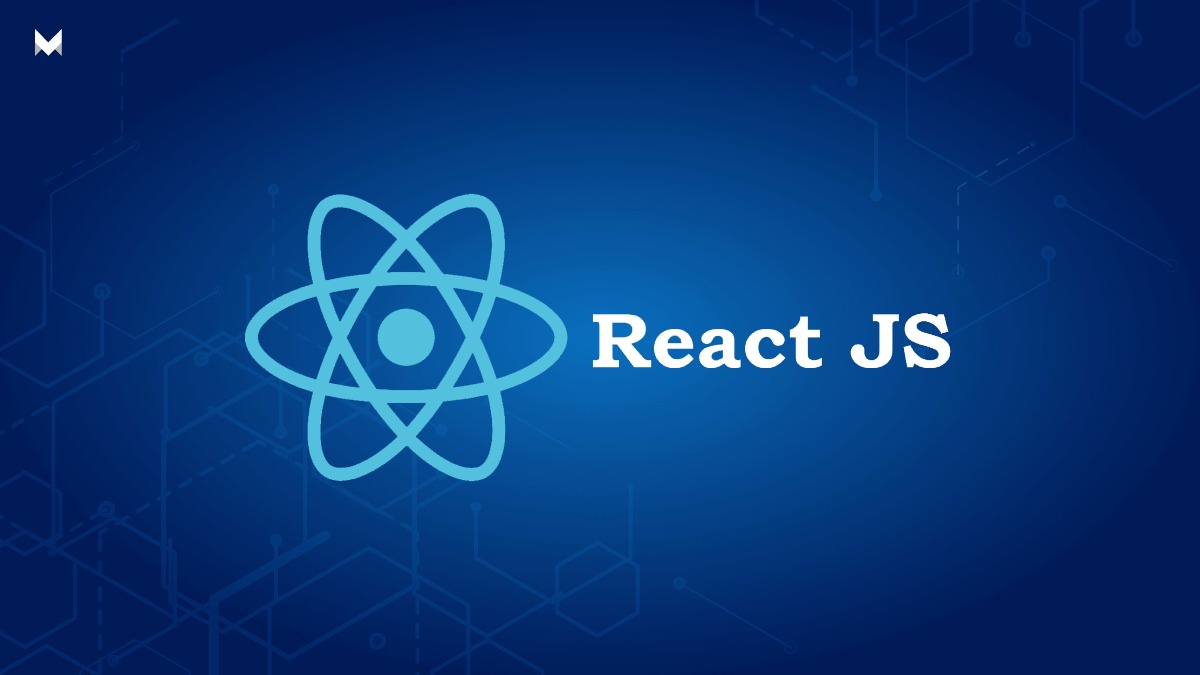
You’ve probably heard of JavaScript frameworks like React, Vue, or Angular if you want to work as a frontend or Mern full stack developer. For the time being, let’s concentrate on React, which is clearly the most popular JavaScript frontend library. To explain the MEVN and MEAN stack, we’ll take a quick look at Vue and Angular. But, for the time being, let’s learn more about React.
As previously said, it is a Facebook library that helps us to efficiently construct dynamic and interactive user interfaces on our websites. In our web application, it uses props (short for properties) and state to provide dynamic interfaces. Furthermore, it allows us to divide down our code into smaller parts, known as components, allowing us to make it more adaptable.
Components may be imported and utilised in numerous locations across our web application, saving us time and energy by avoiding the need to rewrite code for minor changes and keeping our codebase DRY (don’t repeat yourself).
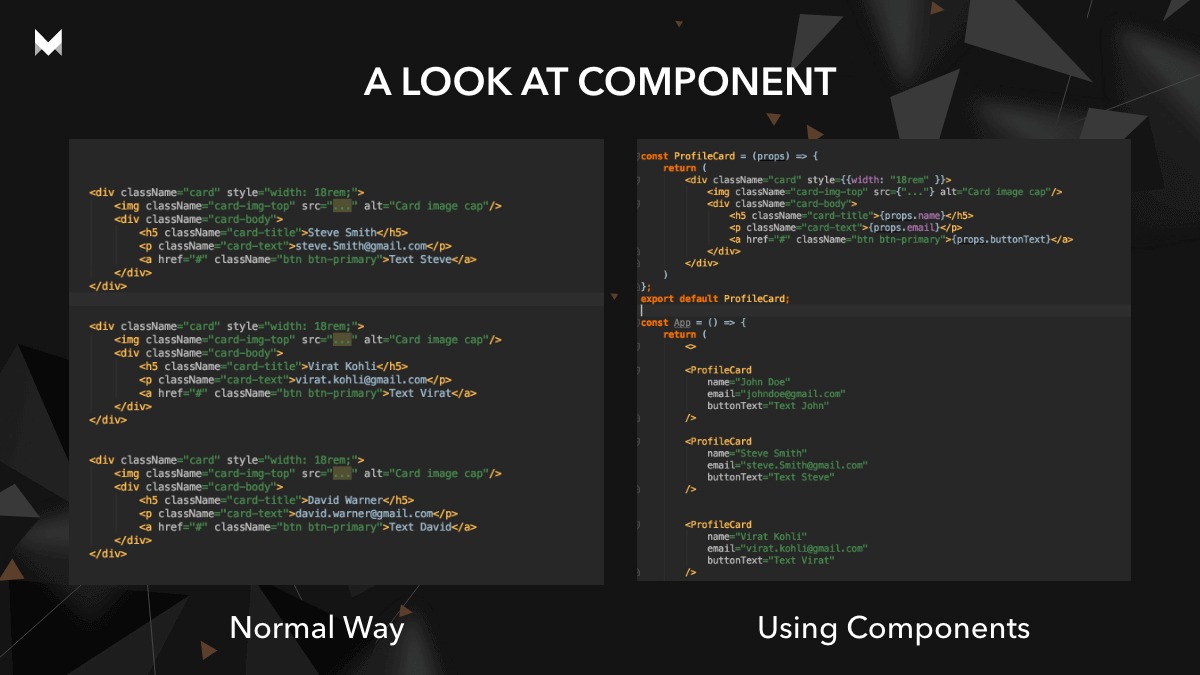
One of the nicest features of React is that it can be used to build single-page apps, or SPAs. In contrast to traditional websites, which reload and retrieve new assets every time you visit a new page of a website, SPAs just require us to load all of our static assets like HTML, CSS, and JavaScript at once. One of the most common ways to implement routing is with react-router-dom. In a nutshell, load once and view the entire website without ever having to refresh, unless you reload manually, of course!
We could write a separate post about React and what makes it so special. Because this is more of a general introduction of the MERN stack than just React, I’ll mention some of the aspects of React that you’ll encounter while creating web apps and should learn:
- Using props and creating suitable components
- Create React code that works useState and useEffect are two often used React hooks.
- Using the useState hook to manage props in a component
- Rendering with conditions
- Using the useEffect hook to make API requests to retrieve data from the backend
- Controlling form inputs and processing form submissions
NodeJS
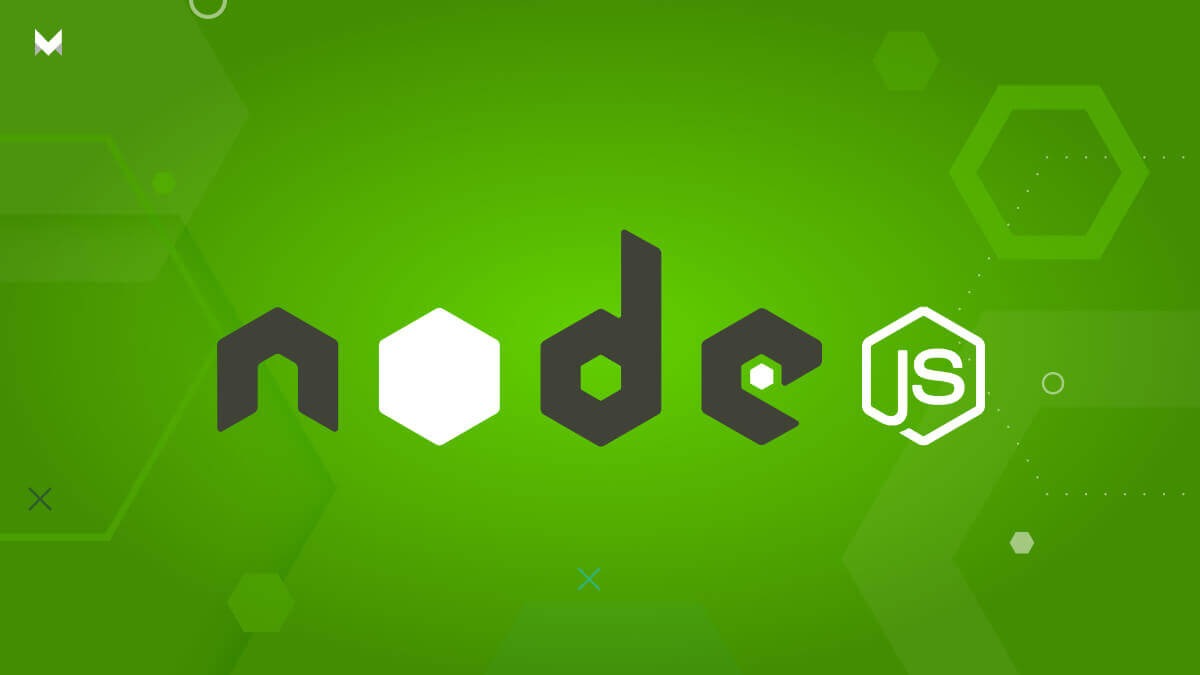
Finally, we’ll look into NodeJS to round up our review of the MERN stack’s technologies. To begin, what exactly is NodeJS and how does it vary from JavaScript? Is it something else entirely; why do we need it in the first place; couldn’t JavaScript be used instead? In the following lines, I’ll attempt to answer all of these often asked questions. But first, let us define NodeJS.
NodeJS is a cross-platform JavaScript runtime environment that runs JavaScript code outside of the browser using Google’s V8 engine. Although JavaScript is designed to run on browsers, we don’t have any on our backend, do we? NodeJS thrives in this area. It allows us to develop JavaScript that can be executed on our backend servers. But how does it manage to do so? It makes use of the V8 engine and libuv, which is a separate issue. In a nutshell, here’s a graphic depiction of how the NodeJS architecture works:
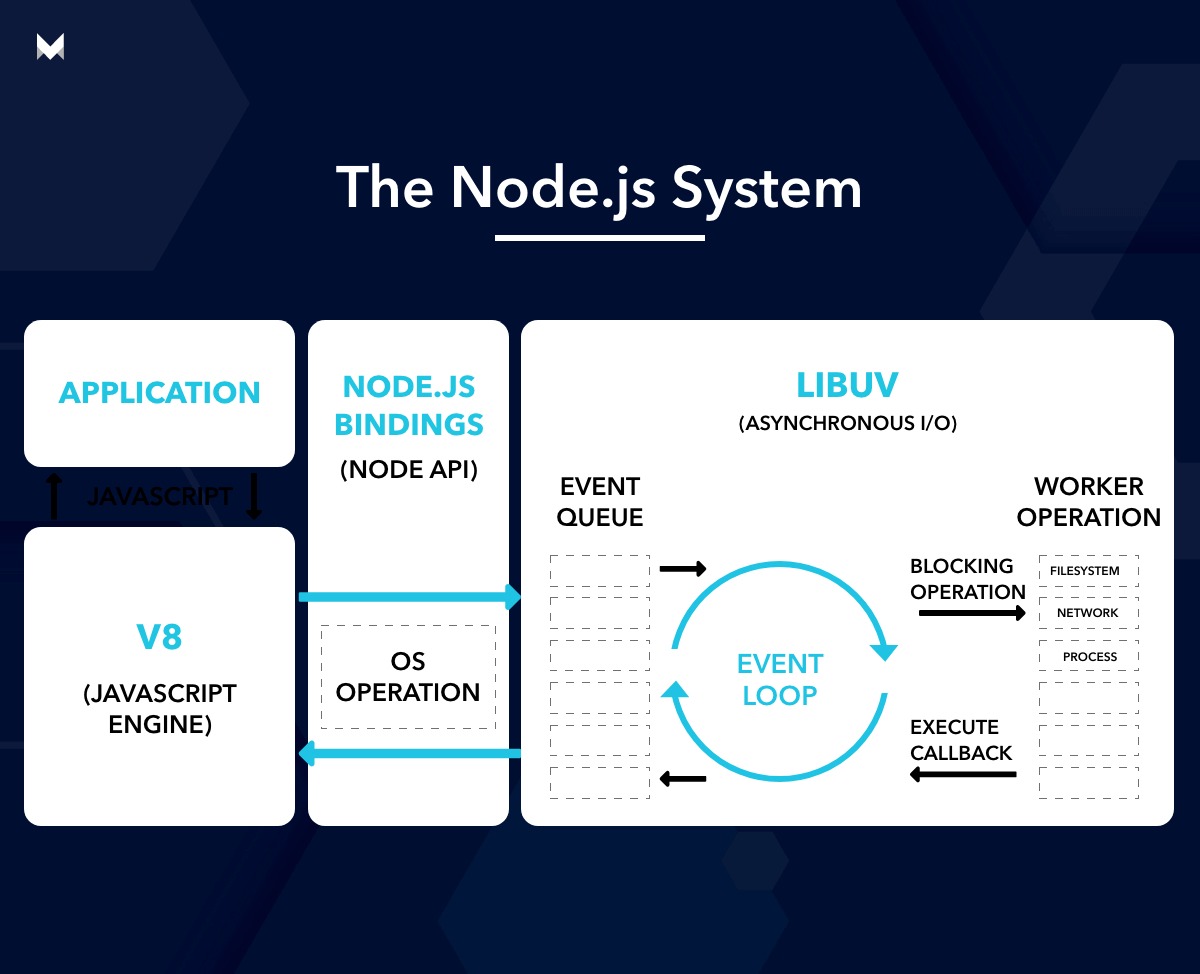
Returning to the MERN stack, NodeJS serves a straightforward purpose. Allow us to develop our backend in JavaScript, sparing us the burden of learning a new programming language. Furthermore, it’s an event-driven, non-blocking I/O paradigm. While there isn’t much unique to NodeJS that you need to know in order to develop a MERN stack application, here are some related resources:
- Initialising a npm package
- Installing npm packages through npm or yarn
- Importing and exports modules using commonJS
- Understanding the package.json file
Combining Technologies and Investigating How They Interact
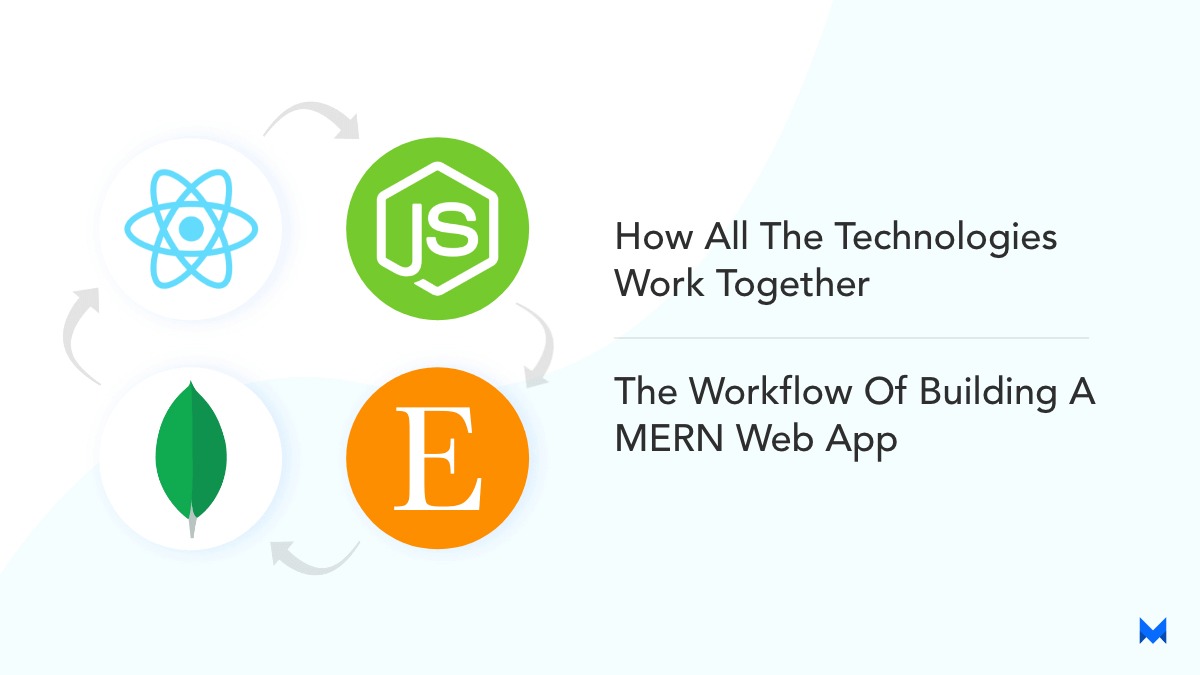
We looked at all four technologies that make up the MERN stack development in the previous section. While each of these technologies is wonderful to work with on its own, integrating them into a web application that runs like clockwork is fantastic. This is what we’ll be learning about in this part. Knowing how to use each technology is half the battle; the other half is piecing it all together into something useful. What could be better than a visual depiction of a MERN stack development web application in which the user interacts with the frontend, which then interacts with the backend and database?
Let’s look at an example to help us comprehend the entire procedure. Assume we’ve created a fantastic e-commerce website that sells clothing. Our website is currently being used by a consumer who is looking for some sneakers. On the landing page, there is a link that sends the user to the shoes page. So, what’s the best way to retrieve data from the backend? Let’s take things slowly at first.
- The user is on our website’s landing page, which was developed with React.
- The user selects the link to go shopping for sneakers. Because we have a single page application, we render the shoes page without refreshing the page.
- At this time, we don’t have any shoe data, therefore the state is empty. To retrieve the data, we issue an API request to our backend.
- Because the process of retrieving data from our database is asynchronous, which means it may take some time to complete, we show the user a loading GIF while the data for shoes is being obtained.
- In the backend, ExpressJS examines the endpoint (route) we’ve reached and calls the relevant controller function to obtain the shoes’ data.
- We utilise mongoose within this controller method to query our database, retrieve the data, and return it in JSON format (JavaScript Object Notation).
- This JSON data is delivered back to our React frontend, where we can use it to update the state with the freshly acquired information.
- React will re-render the components that rely on our state now that it has been changed, so we replace our loading GIF with the shoes information.
Winding Up
As a leading MERN stack development company with a globally-dispersed clientele, Hire MERN Stack developers, from Mobcoder which helps you leverage the powerful capabilities of MongoDB, Express.js, React, and Node.js with reliable, round-the-clock support.